Setup husky 🐕 with Prettier 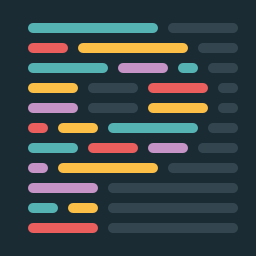
Before installing husky,we have to initiate git in the project by the command
Install the pakage husky as dev dependency by the command
After installing the pakage , add script to the pakage.json
file by performing the command
Now install the prettier pakage by the command -
After installing prettier ,create a file named .prettierrc.json
in the project folder and added the script for prettier rules
{
"trailingComma": "es5",
"tabWidth": 2,
"semi": true,
"singleQuote": true,
"jsxSingleQuote": true,
"bracketSpacing": true
}
prettier will format the code based on these rules.
Now, to run prettier automatically during commit ,we need lint-staged
npm pakage.To install the pakage run the command
Now added the "lint-staged":{}
with the script "*.{js,jsx,ts,tsx,md,html,css}": "prettier --write"
anywhere in the pakage.json
file
Click me to toggle the code
{
"devDependencies": {
"husky": "^9.1.7",
"lint-staged": "^15.2.11",
"prettier": "^3.4.2"
},
"scripts": {
"prepare": "husky"
},
"lint-staged": {
"*.{js,jsx,ts,tsx,md,html,css}": "prettier --write"
}
}
Now run the command
It will create a file named .husky
.Inside the folder there will be a file named pre-commit
.Inside the pre-commit write
npx lint-staged
and saved the file.
Now everything is set up.Execute the commands -
Setup Eslint with Husky 🐕
In the .husky
folder ,that was created before ,inside that folder open the pre-commit file and add the line
npm run lint
Now run the command
The command will execute the lines saved in the pre-commit file.In the terminal you can see the following lines
If there are no errors but warnings present, the code will commit successfully. If you don't want the code to be committed with warnings, you can modify the .json file as shown below:
Click me to toggle the code
{
"devDependencies": {
"husky": "^9.1.7",
"lint-staged": "^15.2.11",
"prettier": "^3.4.2"
},
"scripts": {
"prepare": "husky"
},
"lint-staged": {
"*.{js,jsx,ts,tsx,md,html,css}": [
"prettier --write",
"eslint --max-warnings=0"
]
}
}
"eslint --max-warnings=0"
This line indicates that if there is any warning present in the code,the commit will fail.The codes could not hold any warning also no error for a successful commit.